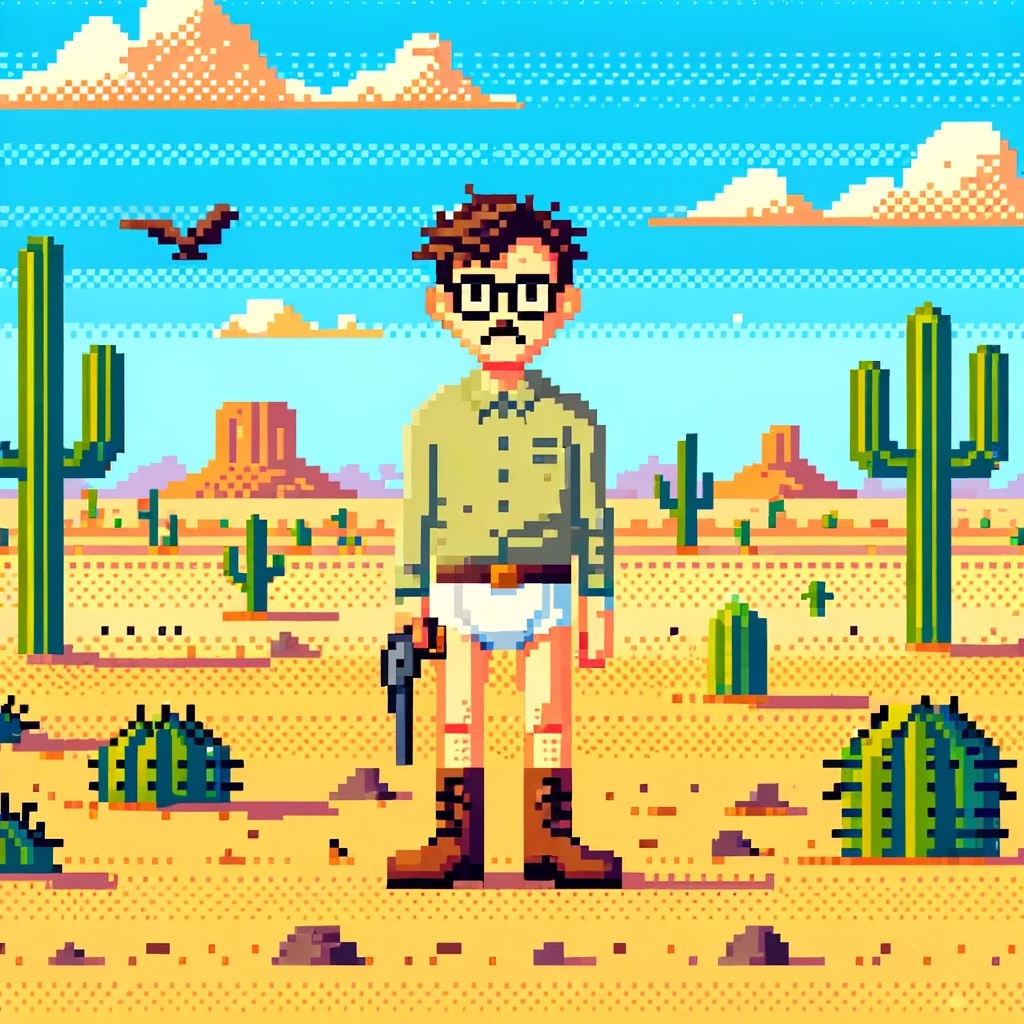
Breaking Solid: Challenges of Adding New Functionality to the Sync Framework
Exploring the Challenges of Adding New Functionality to a Sync Framework: A Balance Between Innovation and SOLID Design Principles
In the evolving landscape of software development, frameworks and systems must adapt to new requirements and functionalities to remain relevant and efficient. One such system, the sync framework, is a crucial component for ensuring data consistency across various platforms. However, introducing new features to such a framework often involves navigating a complex web of design principles and potential breaking changes. This article explores these challenges, focusing on the SOLID principles and the strategic decision-making required to implement these changes effectively.
The Dilemma: Enhancing Functionality vs. Maintaining SOLID Principles
The SOLID principles, fundamental to robust software design, often pose significant challenges when new functionalities need to be integrated. Let’s delve into these principles and the specific dilemmas they present:
Single Responsibility Principle (SRP)
Challenge: Each class or module should have one reason to change. Adding new functionality can often necessitate changes in multiple classes, potentially violating SRP.
Example: Introducing an event trigger in the sync process might require modifications in logging, error handling, and data validation modules.
Open/Closed Principle (OCP)
Challenge: Software entities should be open for extension but closed for modification. Almost any change to a sync framework to add new features seems to require modifying existing code, thus breaching OCP.
Example: To add a new synchronization event, developers might need to alter existing classes to integrate the new event handling mechanism, directly contravening OCP.
Liskov Substitution Principle (LSP)
Challenge: Subtypes must be substitutable for their base types without altering the correctness of the program. Adding new behaviors can lead to subtype implementations that do not perfectly align with the base class, breaking LSP.
Example: If a new type of sync operation is added, ensuring it fits seamlessly into the existing hierarchy without breaking existing functionality can be difficult.
Interface Segregation Principle (ISP)
Challenge: Clients should not be forced to depend on interfaces they do not use. Adding new features might necessitate bloating interfaces with methods not required by all clients.
Example: Introducing a new sync event might require adding new methods to interfaces, which might not be relevant to all implementing classes.
Dependency Inversion Principle (DIP)
Challenge: High-level modules should not depend on low-level modules, but both should depend on abstractions. Introducing new functionalities often leads to direct dependencies, violating DIP.
Example: A new event handling mechanism might introduce dependencies on specific low-level modules directly in the high-level synchronization logic.
Strategic Decision-Making: When to Introduce Breaking Changes
Given these challenges, developers must decide the optimal time to introduce breaking changes. Here are some key considerations:
Assessing the Impact
Evaluate the extent of the changes required and their impact on existing functionality. If the changes are extensive and unavoidable, it might be the right time to introduce a new version of the framework.
Versioning Strategy
Adopting semantic versioning can help manage expectations and communicate changes effectively. A major version increment (e.g., from 2.x to 3.0) signals significant changes, including potential breaking changes.
Deprecation Policies
Gradually deprecating old functionalities while introducing new ones can provide a smoother transition path. Clear documentation and communication are crucial during this phase.
Community and Stakeholder Engagement
Engage with the community and stakeholders to understand their needs and concerns. This feedback can guide the decision-making process and ensure that the changes align with user requirements.
Automated Testing and Continuous Integration
Implement comprehensive testing and CI practices to ensure that changes do not introduce unintended regressions. This can help maintain confidence in the framework’s stability despite the changes.
Conclusion
Balancing the need for new functionality with adherence to SOLID principles is a delicate task in the development of a sync framework. By understanding the inherent challenges and strategically deciding when to introduce breaking changes, developers can evolve the framework while maintaining its integrity and reliability. This process involves not just technical considerations but also thoughtful engagement with the user community and meticulous planning.
Implementing new features is not merely about adding code but about evolving the framework in a way that serves its users best, even if it means occasionally bending or breaking established design principles.