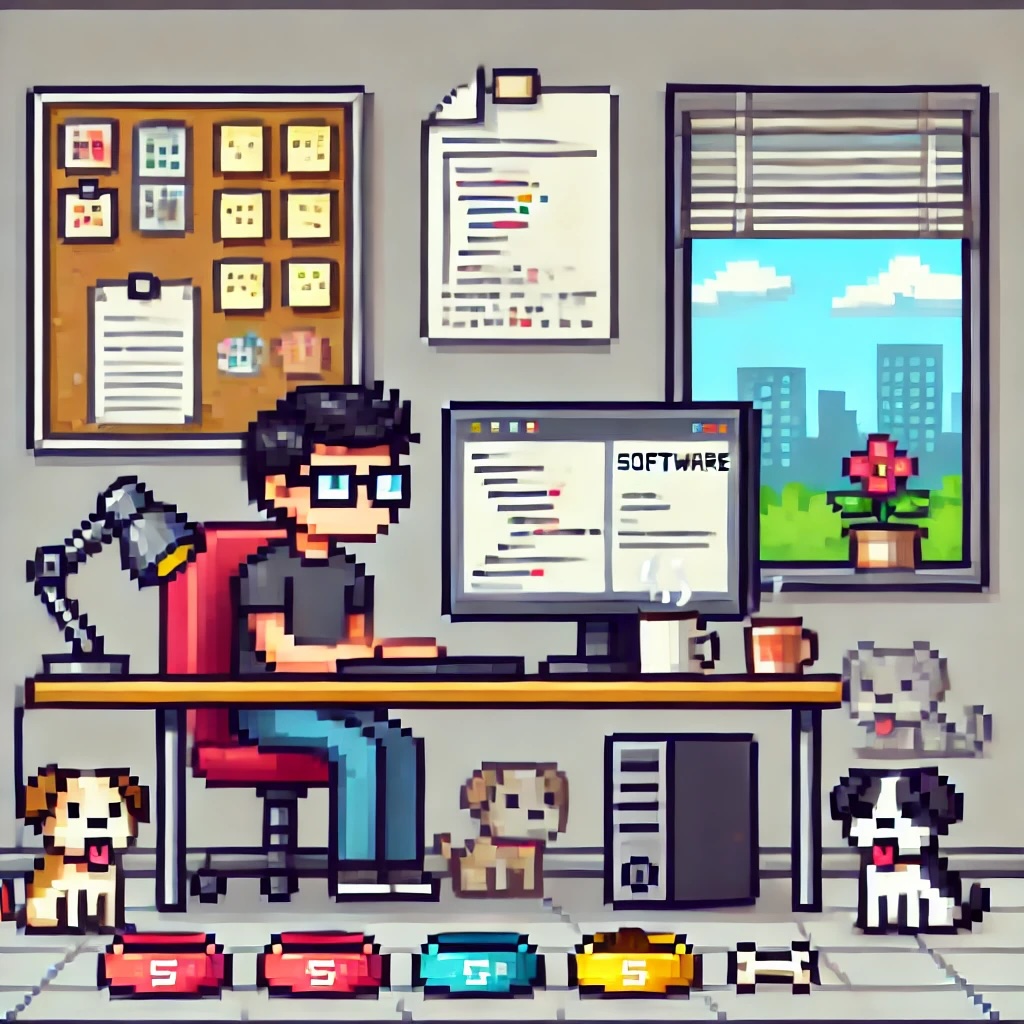
Embrace the Dogfood: How Dogfooding Can Transform Your Software Development Process
Hey there, fellow developers! Today, let’s talk about a practice that can revolutionize the way we create, test, and perfect our software: dogfooding. If you’re wondering what dogfooding means, don’t worry, it’s not about what you feed your pets. In the tech world, “eating your own dog food” means using the software you develop in your day-to-day operations. Let’s dive into how this can be a game-changer for us.
Why Should We Dogfood?
- Catch Bugs Early: By using our own software, we become our first line of defense against bugs and glitches. Real-world usage uncovers issues that might slip through traditional testing. We get to identify and fix these problems before they ever reach our users.
- Enhance Quality Assurance: There’s no better way to ensure our software meets high standards than by relying on it ourselves. When our own work depends on our product, we naturally aim for higher quality and reliability.
- Improve User Experience: When we step into the shoes of our users, we experience firsthand what works well and what doesn’t. This unique perspective allows us to design more intuitive and user-friendly software.
- Create a Rapid Feedback Loop: Using our software internally means continuous and immediate feedback. This quick loop helps us iterate faster, refining features and squashing bugs swiftly.
- Build Credibility and Trust: When we show confidence in our software by using it ourselves, it sends a strong message to our users. It demonstrates that we believe in what we’ve created, enhancing our credibility and trustworthiness.
Real-World Examples
- Microsoft: They’re known for using early versions of Windows and Office within their own teams. This practice helps them catch issues early and improve their products before public release.
- Google: Googlers use beta versions of products like Gmail and Chrome. This internal testing helps them refine their offerings based on real-world use.
- Slack: Slack’s team relies on Slack for communication, constantly testing and improving the platform from the inside.
How to Start Dogfooding
- Integrate it Into Daily Work: Start by using your software for internal tasks. Whether it’s a project management tool, a communication app, or a new feature, make it part of your team’s daily routine.
- Encourage Team Participation: Get everyone on board. The more diverse the users, the more varied the feedback. Encourage your team to report bugs, suggest improvements, and share their experiences.
- Set Up Feedback Channels: Create dedicated channels for feedback. This could be as simple as a Slack channel or a more structured feedback form. Ensure that the feedback loop is easy and accessible.
- Iterate Quickly: Use the feedback to make quick improvements. Prioritize issues that affect usability and functionality. Show your team that their feedback is valued and acted upon.
Overcoming Challenges
- Avoid Bias: While familiarity is great, it can also lead to bias. Pair internal testing with external beta testers to get a well-rounded perspective.
- Manage Resources: Smaller teams might find it challenging to allocate resources for internal use. Start small and gradually integrate more aspects of your software into daily use.
- Consider Diverse Use Cases: Remember, your internal environment might not replicate all the conditions your users face. Keep an eye on diverse scenarios and edge cases.
Conclusion
Dogfooding is more than just a quirky industry term. It’s a powerful practice that can elevate the quality of our software, speed up our development cycles, and build stronger trust with our users. By using our software as our customers do, we gain invaluable insights that can lead to better, more reliable products. So, let’s embrace the dogfood, turn our critical eye inward, and create software that we’re not just proud of but genuinely rely on. Happy coding, and happy dogfooding! 🐶💻
Feel free to share your dogfooding experiences in the comments below. Let’s learn from each other and continue to improve our craft together!