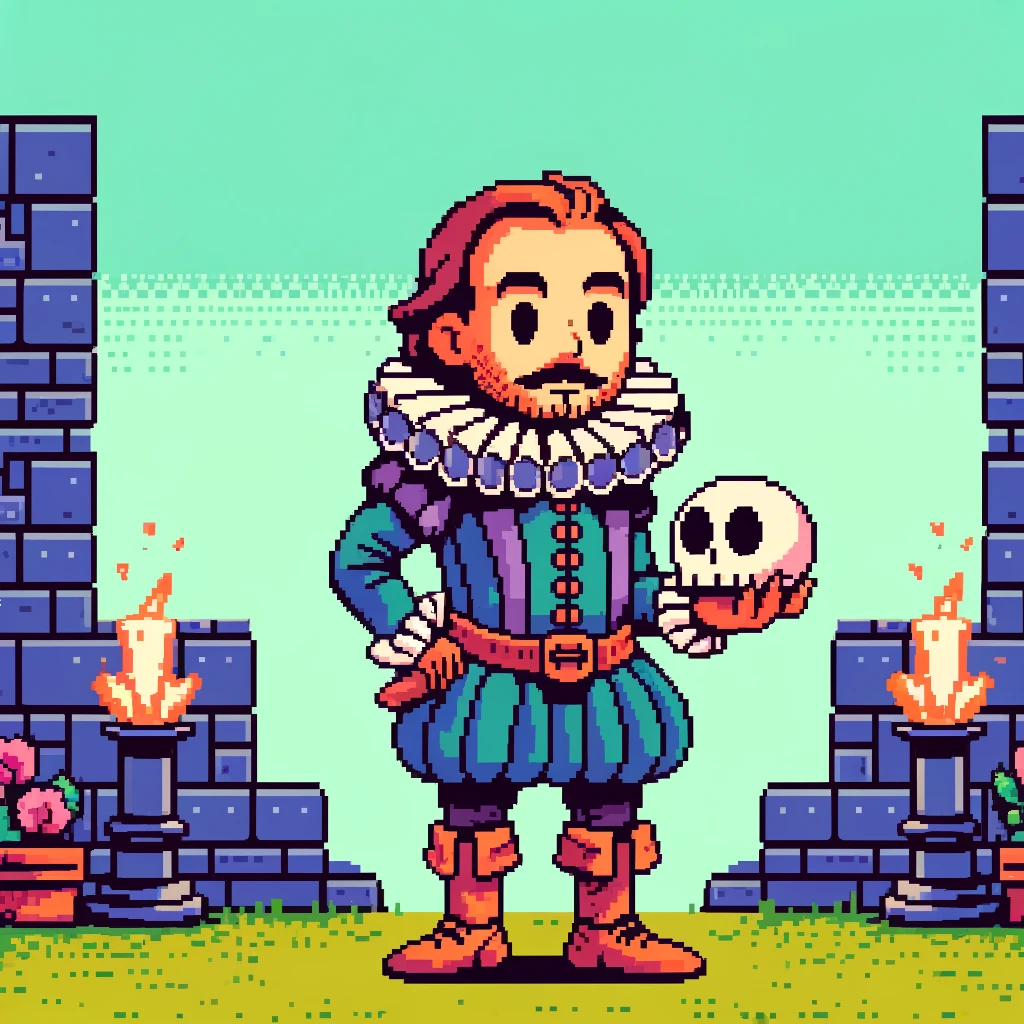
To be, or not to be: Writing Reusable Tests for SyncFramework Interfaces in C#
Writing Reusable Tests for SyncFramework Interfaces in C#
When creating a robust database synchronization framework like SyncFramework, ensuring that each component adheres to its defined interface is crucial. Reusable tests for interfaces are an essential aspect of this verification process. Here’s how you can approach writing reusable tests for your interfaces in C#:
1. Understand the Importance of Interface Testing
Interfaces define contracts that all implementing classes must follow. By testing these interfaces, you ensure that every implementation behaves as expected. This is especially important in frameworks like SyncFramework, where different components (e.g., IDeltaStore
) need to be interchangeable.
2. Create Base Test Classes
Create abstract test classes for each interface. These test classes should contain all the tests that verify the behavior defined by the interface.
using Microsoft.VisualStudio.TestTools.UnitTesting;
public abstract class BaseDeltaStoreTest
{
protected abstract IDeltaStore GetDeltaStore();
[TestMethod]
public void TestAddDelta()
{
var deltaStore = GetDeltaStore();
deltaStore.AddDelta("delta1");
Assert.IsTrue(deltaStore.ContainsDelta("delta1"));
}
[TestMethod]
public void TestRemoveDelta()
{
var deltaStore = GetDeltaStore();
deltaStore.AddDelta("delta2");
deltaStore.RemoveDelta("delta2");
Assert.IsFalse(deltaStore.ContainsDelta("delta2"));
}
// Add more tests to cover all methods in IDeltaStore
}
3. Implement Concrete Test Classes
For each implementation of the interface, create a concrete test class that inherits from the base test class and provides an implementation for the abstract method to instantiate the concrete class.
using Microsoft.VisualStudio.TestTools.UnitTesting;
[TestClass]
public class ConcreteDeltaStoreTest : BaseDeltaStoreTest
{
protected override IDeltaStore GetDeltaStore()
{
return new ConcreteDeltaStore();
}
}
4. Use a Testing Framework
Utilize a robust testing framework such as MSTest, NUnit, or xUnit to ensure all tests are run across all implementations.
5. Automate Testing
Integrate your tests into your CI/CD pipeline to ensure that every change is automatically tested across all implementations. This ensures that any new implementation or modification adheres to the interface contracts.
6. Document Your Tests
Clearly document your tests and the rationale behind reusable tests for interfaces. This will help other developers understand the importance of these tests and encourage them to add tests for new implementations.
Example of Full Implementation
// IDeltaStore Interface
public interface IDeltaStore
{
void AddDelta(string delta);
void RemoveDelta(string delta);
bool ContainsDelta(string delta);
}
// Base Test Class
using Microsoft.VisualStudio.TestTools.UnitTesting;
public abstract class BaseDeltaStoreTest
{
protected abstract IDeltaStore GetDeltaStore();
[TestMethod]
public void TestAddDelta()
{
var deltaStore = GetDeltaStore();
deltaStore.AddDelta("delta1");
Assert.IsTrue(deltaStore.ContainsDelta("delta1"));
}
[TestMethod]
public void TestRemoveDelta()
{
var deltaStore = GetDeltaStore();
deltaStore.AddDelta("delta2");
deltaStore.RemoveDelta("delta2");
Assert.IsFalse(deltaStore.ContainsDelta("delta2"));
}
// Add more tests to cover all methods in IDeltaStore
}
// Concrete Implementation
public class ConcreteDeltaStore : IDeltaStore
{
private readonly HashSet _deltas = new HashSet();
public void AddDelta(string delta)
{
_deltas.Add(delta);
}
public void RemoveDelta(string delta)
{
_deltas.Remove(delta);
}
public bool ContainsDelta(string delta)
{
return _deltas.Contains(delta);
}
}
// Concrete Implementation Test Class
using Microsoft.VisualStudio.TestTools.UnitTesting;
[TestClass]
public class ConcreteDeltaStoreTest : BaseDeltaStoreTest
{
protected override IDeltaStore GetDeltaStore()
{
return new ConcreteDeltaStore();
}
}
// Running the tests
// Ensure to use a test runner compatible with MSTest to execute the tests